Just a quick tip here as it came up in the presentation I did today - how do you handle running code when a particular view runs in Ionic? Let me begin with an example so you get what I'm talking about. Given a new Ionic application using the default tabs application, I'm going to modify the Account controller to add a random number to the scope:
.controller('AccountCtrl', function($scope) {
$scope.settings = {
enableFriends: true
};
console.log("Running stuff...");
$scope.number = Math.floor(Math.random()*10);
})
In the view for this view I added a quick display for the number: Random number: {{number}}
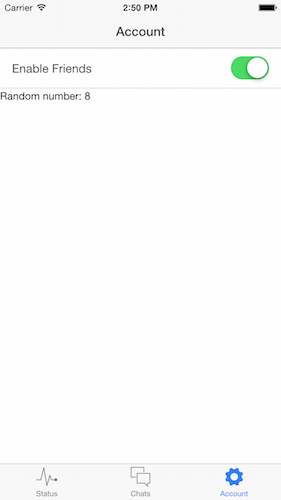
Yeah, not the most exciting demo, but you get the idea. What you'll notice right away though is that as you click back and forth between different tabs, the value doesn't change. And if you open up your remote dev tools, you'll see the console message run only once.
This is due to the default caching system built into the ion-view directive. Luckily it is rather easy to fix with one of the life cycle events. The docs say you can listen for these events but don't demonstrate how. Here is an example:
.controller('AccountCtrl', function($scope) {
$scope.settings = {
enableFriends: true
};
$scope.$on("$ionicView.beforeEnter", function() {
console.log("Running stuff...");
$scope.number = Math.floor(Math.random()*10);
});
})
And that's it. For me the tricky part was $scope.$on, something I've not used in Angular before. You can read more about the events at the docs.
Archived Comments
Hey ray, I have been wondering how i could handle running code when a particular view runs in Ionic. You nailed it in this post. thanks
I just noticed found the same thing yesterday when I created a new app using the tab template, it had commented out code in the chats controller for this. Didn't even know about this feature. So many good features it is hard to keep up on all of them.
Yeah, I need to read the Ionic docs from start to finish one day soon.
strange today on IOS beforeEvent is not fired when clicking href="#/app/mystate" ... instead it works if $state.go() is used :-(
Could be a chance in Ionic since I posted this. Thanks for sharing though.
nevermind i found the problem, another $stateParams was sharing the same URL with a different controller :) So "#/app/loading" goes to the 1st element of stateParam's config while $state.go() goes to the 2nd one... LOLz ???
I like Angular, but sometimes, yeah, it is a bit weird.
It helped !! thanks for such a brief and helpful article.
thanks a lot..was looking for a workaround