Well, this was a weird one. Yesterday I was working on a project where I made multiple requests to the Random User API. It worked perfectly in Chrome, and Android, but in Safari, I noticed something odd. Even though I made multiple requests, every result was the same, not unique. Here is a simplified version of what I built.
for(var i=0;i<10;i++) {
$.ajax({
url: 'http://api.randomuser.me/',
dataType: 'json',
success: function(data){
console.log(JSON.stringify(data.results[0].user.name));
}
});
}
My code was somewhat more complex (it had Angular, Promises, even kittens thrown in), but this gives you the basic idea. Running this in Chrome I get what I'd expect, 10 random users in the console:
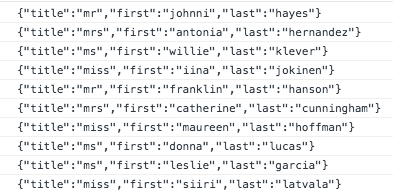
And ten requests in the Network panel:
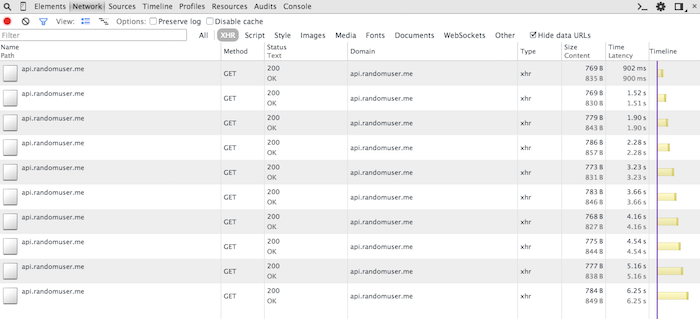
So far so good. However, in Safari (well, Mobile Safari at first, but today I tested in Safari), something odd happened. Instead of ten random users, I got the same one again and again. (And before someone asks, no, it isn't the for loop or anything like that.)
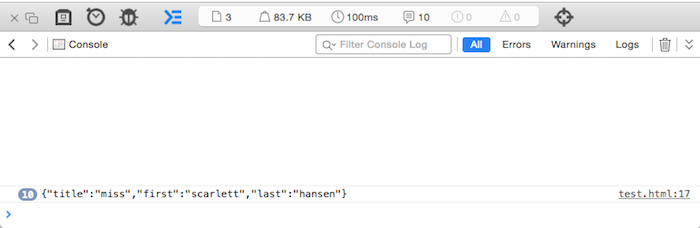
Naturally I thought - ok - Safari is caching the response. But here is what threw me for a loop. I went into the Timelines panel, turned on Recording, and this is what I saw:
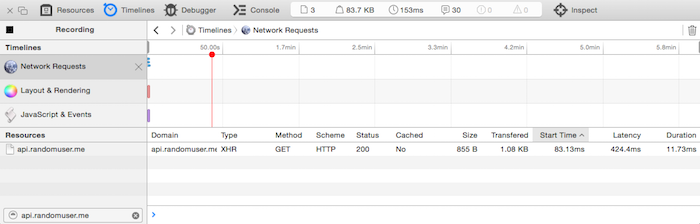
Looking at this, you can see Safari made one network request, which I suppose makes sense, but here is what ticks me off. Nowhere in this panel is any indication that it simply ignored my Ajax calls and used a cache result.
To be clear, I'm totally fine with Safari ignoring my request to an API that is random and deciding it knew better and should cache. Fine. What upsets me is that the dev tools do zero to let the developer know what's going on here. It should report the other 9 requests and flag them as being from a cache or some such. I guess I'll go file a bug report for this (no, I will, because that's the right thing to do), but damn was this frustrating.
For folks curious, I simply added "?safaricanbiteme="+Math.random() to the URL - just like I used to do for older IE.