(As just an FYI, while working on this demo I discovered the Analytics Embed API. It looks like a powerful way to create Google Analytics mashups and I plan on looking at it deeper in the next week or so. Note that the code I use here could be much simpler with the Embed API.)
This past fall I switched from a custom blog engine I had written to Wordpress. While in general I'm really happy with the move, there's one thing my old blog had that I really miss. Whenever I logged into the admin, I'd see my last five blog posts and how many views they had received so far. For me, this was a great way to gauge how my current articles were doing. I obviously checked my Google Analytics often as well, but the report of my current entries was a great way to see how my most recent articles were being received.
A year ago I worked on a Google Analytics dashboard that made use of their client-side API. This dashboard simply gave me a birds-eye view of all my properties and how well they are doing. This demo gave me the basics of working with both authentication and the Analytics API, so I began working on a new mashup. The idea was simple:
- Authenticate the user to Google.
- Let them select a property.
- Let them enter a RSS URL that matched the property.
- Use the RSS entries as queries against the Analytics API and report the stats.
In order to make this work, I needed the Core Reporting API and the Feed API. The Feed API actually supports a method of searching for a RSS feed, but it oddly didn't return the RSS feed itself - just matched entries. Because of this my tool needs to ask you for the RSS URL. Before digging into the code, let me share some screen shots so you get an idea of how it looks.
First - the application recognizes if you've recently authenticated with Google. If you have not, it will prompt you to:
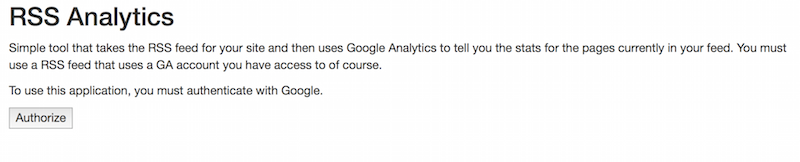
Clicking that button pops open a new window where you'll begin the authentication/authorization process with Google. I've set up a project for this demo and specified what scopes my demo needs. This is what controls the list of requests seen here.
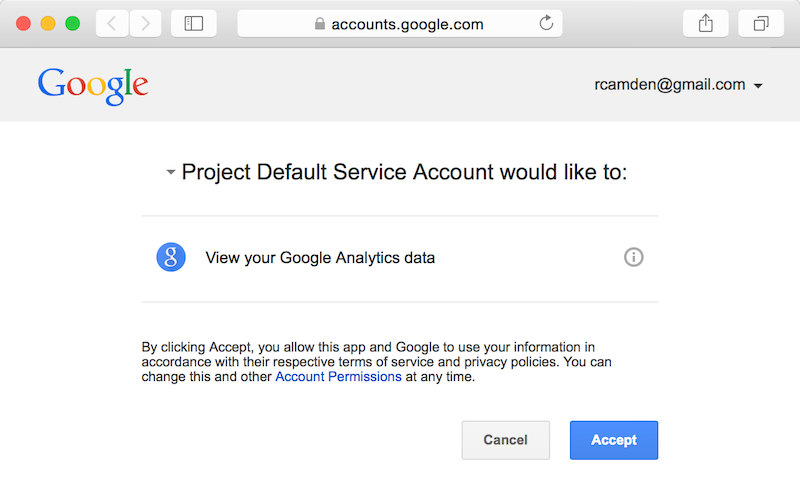
After you've authenticated, the code then uses the Analytics API to fetch the web sites you have access to for that account:
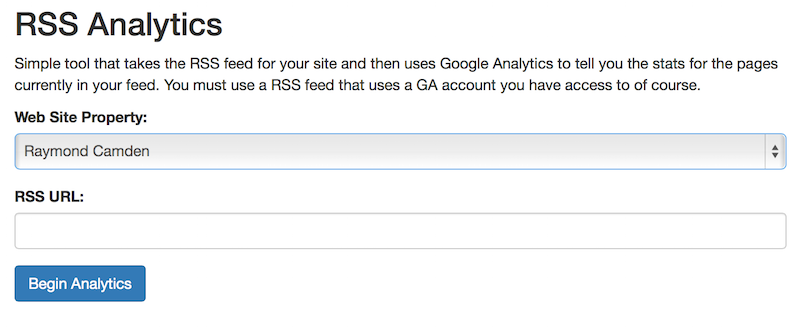
Notice how the RSS field is empty. I added code so that once you enter a value here it will remember it. Next time you load the tool and select your property, you won't need to fill in the values again.
Anyway, once you do enter the value, the Feed API takes over. It parses the RSS and gets your most recent entries.
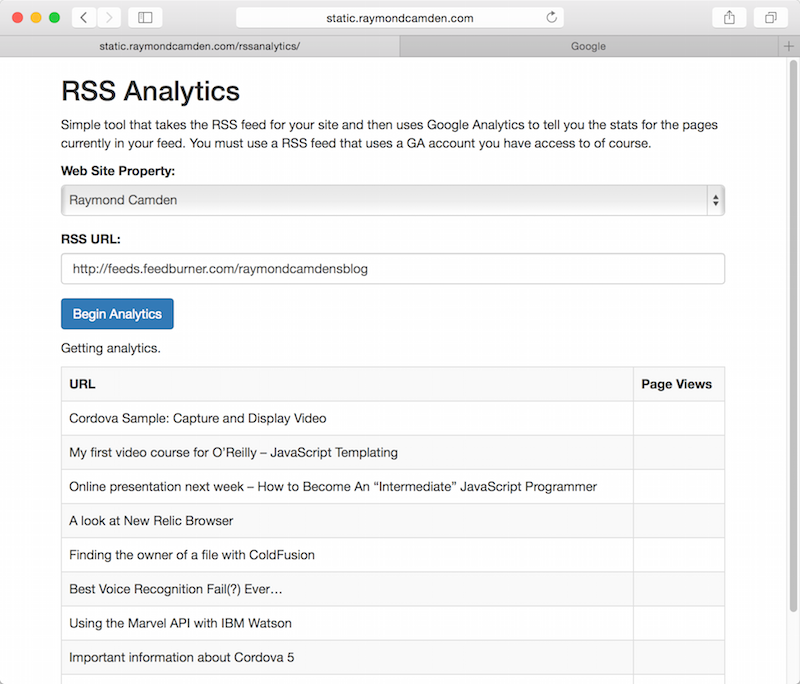
And then magically - the stats show up in the table:
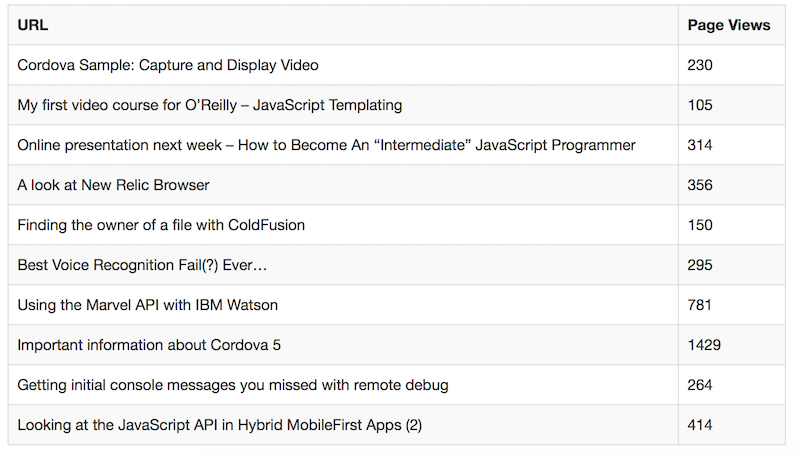
Ok, so how does it work? I won't go over the authentication part as I used the same code from my previous demo. The analytics part was a bit different. I moved it into a module pattern and exposed two APIs - getProperties and getStatsForPropertyURL. getProperties returns a promise which makes using it somewhat simple:
analyticsModule.getProperties().then(function(props) {
//boring DOM crap here
});
}
Behind that simple call though was some complex rejiggering of the previous demo. I make use of LocalStorage to cache values and the Analytics stuff is multi-step, so it took me a few iterations to get it right. Getting stats for a URL was pretty simple. Given you know the property ID and the URL path (you don't include the full URL), here is the call:
function getStatsForPropertyURL(propId, url) {
var adef = $.Deferred();
gapi.client.analytics.data.ga.get({
'ids': 'ga:' + propId,
'start-date': '2005-01-01',
'end-date': 'today',
'metrics': 'ga:pageviews',
'filters':'ga:pagePath=='+encodeURI(url),
'dimensions1':'ga:date'
}).execute(function(results) {
console.dir(results);
adef.resolve(results.totalsForAllResults["ga:pageviews"]);
});
return adef;
}
Note the use of 2005-01-01 as the start date. This is the earliest date that the API supports.
So, want to check it out? Remember - you must have a Google Analytics account and the property you want to test must use a RSS feed. I tested in Chrome, Firefox, and Safari and it seemed to work well, but obviously you can let me know (via an issue or comment) how it works for you:
https://static.raymondcamden.com/rssanalytics/
Want to see all the code, or report an issue? I created a Github repo: https://github.com/cfjedimaster/Google-Analytics-RSS-Parsing