Just a quickie - a user on Stackoverflow asked how to both capture video and display it in the app. This is fairly easy with the Media Capture API so I thought I'd whip up a quick demo.
First, I created an HTML page with a button and an empty div to hold the video later on:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title></title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width">
<link rel="stylesheet" type="text/css" href="css/app.css" />
</head>
<body>
<button id="takeVideo">Take Video</button>
<div id="videoArea"></div>
<script src="cordova.js"></script>
<script src="js/app.js"></script>
</body>
</html>
And then I wrote up the following JavaScript:
document.addEventListener("deviceready", init, false);
function init() {
document.querySelector("#takeVideo").addEventListener("touchend", function() {
console.log("Take video");
navigator.device.capture.captureVideo(captureSuccess, captureError, {limit: 1});
}, false);
}
function captureError(e) {
console.log("capture error: "+JSON.stringify(e));
}
function captureSuccess(s) {
console.log("Success");
console.dir(s[0]);
var v = "<video controls='controls'>";
v += "<source src='" + s[0].fullPath + "' type='video/mp4'>";
v += "</video>";
document.querySelector("#videoArea").innerHTML = v;
}
So I begin by simply listening for a touch event to the button I create. I then use the Media Capture API to start the video process. In the success handler I just take the result's fullPath property and put it in HTML. I was kinda surprised the path worked as I had thought I'd need to use a URL form, but it worked just fine.
Here it is running on my iPad.
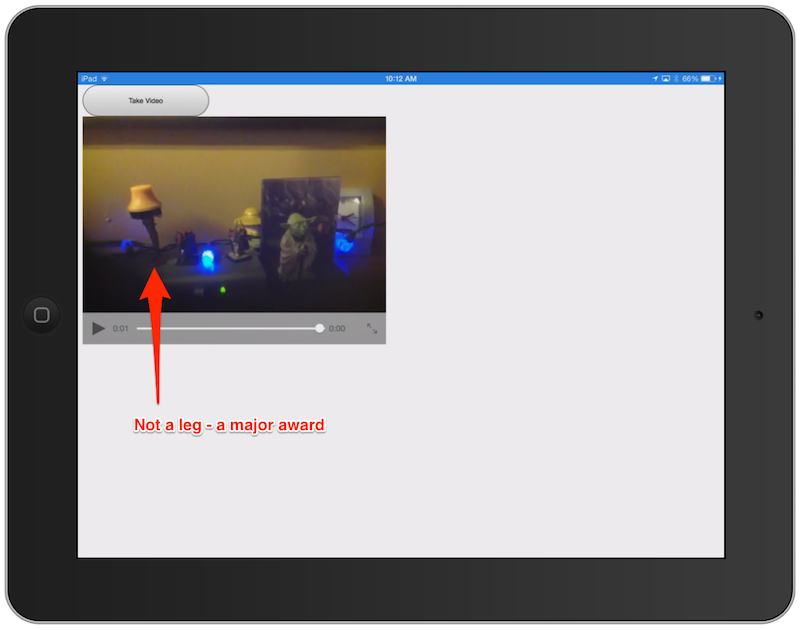
If for some reason you want to try this yourself, I uploaded it to my Cordova demo repo: https://github.com/cfjedimaster/Cordova-Examples/tree/master/videotest1.