Yesterday a reader contacted me asking for help sending SMS messages from a PhoneGap/Cordova application. I've made use of a nice plugin for this before so I thought I'd whip up a quick example of it for him, and my readers.
The plugin I used is Sms Custom Cordova Plugin - not the most imaginative name but really darn simple to use. It has one method, sendMessage, that - wait for it - will send a SMS message. On Android this is done completely behind the scenes. In other words, it will send a SMS message without letting the user know. Obviously you shouldn't do that, but keep that in mind. On iOS and Windows it will actually open the SMS application so the user has to actually finish the process themselves. Let's look at a simple example. As with my other Cordova examples, this is all up on GitHub for you to play with.
First, the HTML. This application does one thing - prompt for a telephone number and message. To make it a tiny bit prettier I added Ratchet.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title></title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width">
<link rel="stylesheet" type="text/css" href="css/ratchet.min.css" />
<script src="js/ratchet.min.js"></script>
</head>
<body>
<header class="bar bar-nav">
<h1 class="title">SMS Demo</h1>
</header>
<div class="content">
<div class="content-padded">
<form>
<input type="text" id="number" placeholder="Phone Number"><br/>
<textarea id="message" placeholder="Message"></textarea>
<input type="button" class="btn btn-positive btn-block" id="sendMessage" value="Send Message">
</form>
</div>
</div>
<script src="cordova.js"></script>
<script src="js/app.js"></script>
</body>
</html>
Which renders this lovely UI:
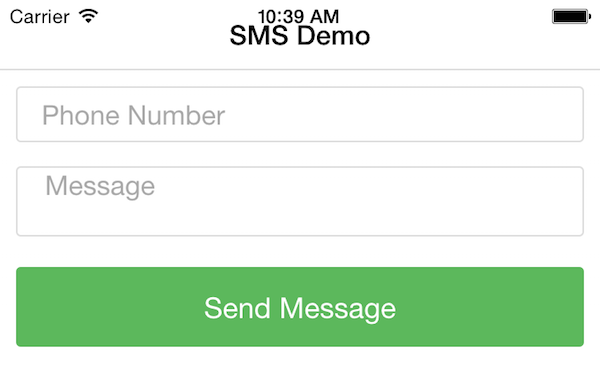
Now let's consider the JavaScript:
document.addEventListener("deviceready", init, false);
function init() {
document.querySelector("#sendMessage").addEventListener("touchend", function() {
console.log("click");
var number = document.querySelector("#number").value;
var message = document.querySelector("#message").value;
console.log("going to send "+message+" to "+number);
//simple validation for now
if(number === '' || message === '') return;
var msg = {
phoneNumber:number,
textMessage:message
};
sms.sendMessage(msg, function(message) {
console.log("success: " + message);
navigator.notification.alert(
'Message to ' + number + ' has been sent.',
null,
'Message Sent',
'Done'
);
}, function(error) {
console.log("error: " + error.code + " " + error.message);
navigator.notification.alert(
'Sorry, message not sent: ' + error.message,
null,
'Error',
'Done'
);
});
}, false);
}
So as I mentioned above, the plugin has one method. It takes an object containing the phone number and message. The second argument is the success handler and the third is for failures. If you try to run this on the simulator, you will get an error: "SMS feature is not supported on this device." This is useful in case your application is being run on a wifi-only tablet.
As I mentioned, on iOS and Windows Phones, the user will see the 'real' SMS application pop up. It will be pre-populated with the data sent from the plugin. Here is an example:
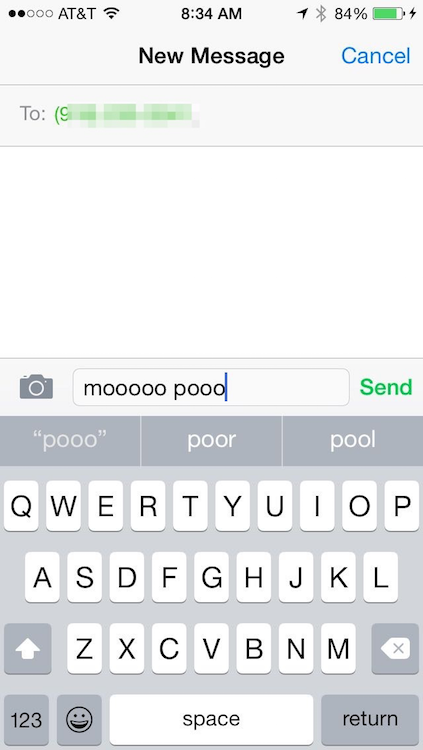
The user will need to hit Send to finish the process, but they won't have to actually type anything.
That's it. If you want a copy of this code, you can grab it here: https://github.com/cfjedimaster/Cordova-Examples/tree/master/smscomposer