This weekend I was reading an email on my phone when I noticed something odd. The link, to a Wikipedia article, prompted me to ask if I wanted to load it in my browser, or in the Wikipedia app. Knowing that the Wikipedia app was built with PhoneGap, I decided to dig into this and see how it was done.
I did some research and discovered that (as far as I could tell), the Wikipedia app was making use of an Android feature called Intents. From my understanding, Intents are a way for applications to...
- Broadcast out a general request - ie, "I have an address and I'd love for someone to do something fancy with it!"
- Listen for general requests - ie, "Dude, I can so do awesome things with maps. If you have an address, let me know, cuz I'll so do something awesome with it. Awesome."
Turns out there is already a plugin for this: WebIntent. To make use of this plugin, you have to modify the Java code a bit (I forgot to bookmark it, but someone else provided the help here) to support the latest version of PhoneGap. I've included a copy with my blog entry so feel free to just copy it from there. But once you have the plugin installed, you can do either (or both) of the actions above.
Creating an intent is as simple as using a bit of JavaScript:
But making your application listen for an intent involves a bit more work, specifically, modifying your AndroidManifest.xml file. You need to add a bit of XML in this to register the intent while also using JavaScript in your application to notice when your app was launched.
Via Stackoverflow, I found this entry on listening out for Youtube links, and then added it to my AndroidManifest.xml:
And then I used a bit of JavaScript to notice the intent.
Simple, right? After installing the application, I whipped up a quick HTML page with two links. One pointing to my blog, another to a random Youtube video.
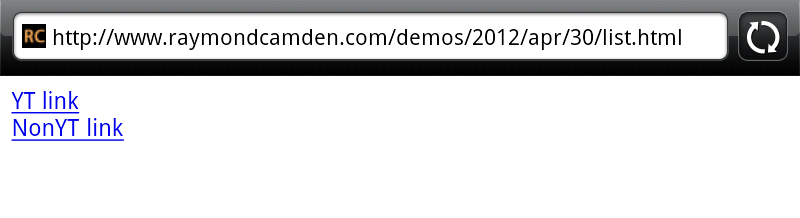
When I click the Youtube link, I now get this:
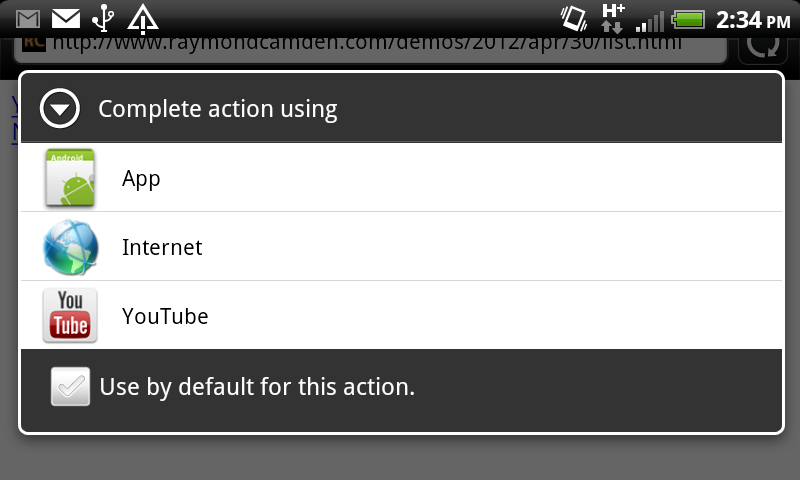
and if I select my own application, the JavaScript I wrote notices and responds to the invocation:
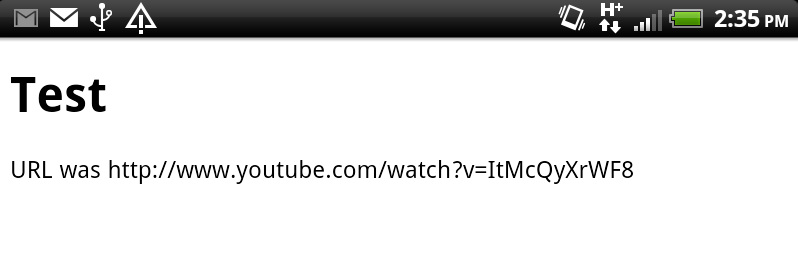
Pretty simple! I really barely touched on the power and reach of Intents, and I have no idea if something similar exists for iOS (surely it must), but all in all this is incredibly easy to use with PhoneGap.