Back in June I wrote a quick blog entry on using the Server Monitor API. Most folks don't know that the cool Server Monitor in ColdFusion 8 is all driven by an underlying CFC API you can use in your own applications. I wrote up a quick demo of this I thought folks might find interesting.
One of the API calls you can make is getRequestDetails. This returns a (possibly) large array of data about each and every single request your site has served up. Requests are organized into array items by template. You get quite a bit of information. The last time the request was made. How many times it erred. When the last error occurred. The last, average, min, and max request times. Etc. With this in mind I wrote up a file that would grab all requests that were last run in the past 3 minutes. My server is busy so this gave a good result set. You may have to tweak that number for your own box. Here is the code:
<cfset password = "parishiltonismylostlovechild">
<cfinvoke component="CFIDE.adminapi.administrator" method="login" adminpassword="#password#" returnVariable="result">
<cfif not result>
<cfabort />
</cfif>
<cfinvoke component="CFIDE.adminapi.servermonitoring" method="getRequestDetails" returnVariable="areq">
<cfset data = queryNew("template,function,timeran,lastresponsetime,avgtime,hitcount,errorcount,lasterror,lasterrorat","varchar,varchar,date,integer,integer,integer,integer,varchar,date")>
<cfloop index="req" array="#areq#">
<!--- only use if within past 3 mins --->
<cfif dateDiff("n", req.lasttimeexecuted, now()) lte 3>
<cfset queryAddRow(data)>
<cfset querySetCell(data, "template", replace(req.templatepath,"","/","all"))>
<cfset querySetCell(data, "function", req.functionname)>
<cfset querySetCell(data, "timeran", req.lasttimeexecuted)>
<cfset querySetCell(data, "lastresponsetime", req.lastresponsetime)>
<cfset querySetCell(data, "avgtime", req.avgtime)>
<cfset querySetCell(data, "hitcount", req.hitcount)>
<cfset querySetCell(data, "errorcount", req.errorcount)>
<cfset querySetCell(data, "lasterror", req.lasterror)>
<cfset querySetCell(data, "lasterrorat", req.lasterrorat)>
</cfif>
</cfloop>
I begin by authenticating with the adminapi using my ColdFusion Administrator password. Then I call the ServerMonitor CFC. The result is an array, so I loop over it, and for each item, I check the date of the last hit. If within the last three minutes, I take out some of the values and stuff them into a query. (I'll explain the replace function in a minute.)
Next I sort the items by time:
<!--- sort by time --->
<cfquery name="data" dbtype="query">
select *
from data
order by timeran desc
</cfquery>
And then I pass to a cool HTML grid:
<cfform name="throwaway">
<cfgrid format="html" query="data" name="requests" width="100%">
<cfgridcolumn name="template" header="Name" width="400">
<cfgridcolumn name="lastresponsetime" header="Last Response Time" width="200">
<cfgridcolumn name="avgtime" header="Avg Response Time" width="200">
<cfgridcolumn name="hitcount" header="Times Ran">
<cfgridcolumn name="errorcount" header="Error Count">
<cfgridcolumn name="lasterror" header="Last Error" width="400">
<cfgridcolumn name="lasterrorat" header="Last Error Occured" width="200">
</cfgrid>
</cfform>
Here is a screen shot. Click for a large version. I hid my physical paths:
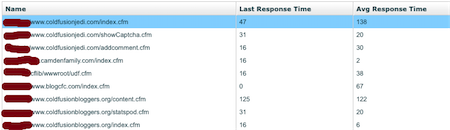
The reason for the replace is to handle the bug I blogged about a few days ago.
Tomorrow I'll demonstrate another example of using the API. Enjoy.
Archived Comments
We're actually having all kinds of trouble getting Server Monitor up and running. Got any tips? Basically, the movie loads, but nothing ever appears on the screen. Several people said they've solved the problem, but never post any details :(
but you have to have enterprise version of CF8
Ray, I've tried your sample code, but I get the following error:
The getAdminHash method was not found.
Either there are no methods with the specified method name and argument types, or the getAdminHash method is overloaded with argument types that ColdFusion cannot decipher reliably. ColdFusion found 0 methods that matched the provided arguments. If this is a Java object and you verified that the method exists, you may need to use the javacast function to reduce ambiguity.
What's interesting is this:
ERROR!! - struct
COLUMN 0
ID CF_STRUCTBEAN
LINE 29
RAW_TRACE at cfadministrator2ecfc1165008047$funcLOGIN.runFunction(C:\blackstone_updates\cfusion\wwwroot\CFIDE\adminapi\administrator.cfc:29)
TEMPLATE C:\blackstone_updates\cfusion\wwwroot\CFIDE\adminapi\administrator.cfc
TYPE CFML
C:\blackstone_updates is not a folder that exists on my server. Any ideas?
Thanks as always
Glen - sounds like maybe a bad Flash client install.
Will - Odd - that call is standard CF Admin API type stuff.
with the blackstone thing, try checking your neo-runtime.xml config file and see if it's pointing to that directory instead of your webroot. Failing that, possibly try searching (via eclipse or textpad or some such thing) all the XML files under your cf install root for "blackstone".
I've run into several of these weird pathing things on cf8, usually pointing to the "e" drive, and it always ended up being a config file that, for whatever reason, had the wrong info.
Good luck!
Hmmm, no mention of that directory in the neo-runtime.xml file, and no mention in any of the files in the cf install root either.
Very strange.
Michael -
We do have the enterprise version of CF8, so that's ruled out.
Ray -
Only if we have a bad Flash client install on 6 different machines.
I'm going to try some other things as well, but at this point, it looks like server monitor is a no go... :(
The specific error we're getting trying to load up the swf:
Either the Macromedia application server(s) are unreachable or none of them has a mapping to process this request.
Glen - at this point, I'd call up Adobe support.
Hi
I got all that Blackstone stuff up as well when being unable to access the coldfusion administrator in CF MX7, although the server itself otherwise worked OK and served up my own pages with no problem - just not the administrator.
Also other key tags (for other's benefit):
The error occurred in Application.cfm: line 113
The web site you are accessing has experienced an unexpected error
So for others who can't access the coldfusion adminstrator, it might be:
See if the file component.cfc is in the wwwroot/web-inf/cftags/ directory of your Coldfusion installation. It is zero bytes - they can get removed by system cleaners (like mine did!) If it's not there, just create a file with that name in notepad or something - with nothing in it, just save it as a filename in the specified directory.
This was driving me crazy!